Master The Art Of Sorting Data In Descending Order With Java
How can we sort elements in descending order using Java?
Sorting in descending order means arranging the elements of a collection in decreasing value. In Java, we can sort an array or a list in descending order using the sort() method and passing a Comparator that specifies the sorting order. For example:
int[] numbers = {5, 2, 8, 3, 1};Arrays.sort(numbers, Collections.reverseOrder());// Output: [8, 5, 3, 2, 1]
Sorting in descending order can be useful in various scenarios, such as finding the largest elements in a dataset or sorting a list of objects by their weight or price.
In addition to the sort() method, Java provides other methods for sorting collections, including the sorted() method, which returns a sorted view of the collection, and the max() and min() methods, which return the maximum and minimum elements, respectively.
Sort in Descending Order in Java
Sorting in descending order is an essential technique for organizing data in Java. It involves arranging elements in decreasing value, from the largest to the smallest.
- Simplicity: Java provides built-in methods like sort() and Collections.reverseOrder() for easy implementation.
- Efficiency: Sorting algorithms in Java, such as quicksort and mergesort, offer efficient time complexity.
- Flexibility: Sorting can be applied to arrays, lists, and other data structures, providing versatility.
- Data Analysis: Descending order sorting helps identify maximum values, outliers, and trends in datasets.
- Optimization: It enables efficient retrieval of largest elements, optimizing performance in applications like priority queues.
- Real-World Applications: Descending order sorting finds use in various domains, including finance, inventory management, and scientific computing.
These aspects highlight the importance of sorting in descending order in Java, making it a valuable technique for data manipulation and analysis.
Simplicity
Sorting in descending order in Java is made simple with the availability of built-in methods like sort() and Collections.reverseOrder(). The sort() method sorts the elements of an array or list in ascending order by default. However, by passing the Collections.reverseOrder() comparator to the sort() method, we can easily sort the elements in descending order.
- Convenience: Collections.reverseOrder() provides a straightforward way to reverse the sorting order without the need for complex coding or custom comparators.
- Efficiency: The built-in sort() method utilizes efficient sorting algorithms like quicksort or mergesort, ensuring optimal performance even for large datasets.
- Clarity: Using the sort() method with Collections.reverseOrder() enhances code readability and maintainability, making it easier to understand and modify the sorting logic.
- Consistency: Java's sorting methods follow consistent naming conventions and behavior across different data structures, simplifying the learning curve and reducing the risk of errors.
In summary, the simplicity and ease of use offered by Java's built-in sorting methods make it a compelling choice for sorting in descending order, contributing to the overall efficiency and clarity of Java programming.
Efficiency
The efficiency of sorting algorithms is a crucial aspect of "sort in descending order java" because it directly impacts the performance and scalability of the sorting operation. Quicksort and mergesort are two widely recognized sorting algorithms in Java known for their exceptional time complexity.
Quicksort utilizes a divide-and-conquer approach, recursively partitioning the input array into smaller subarrays until it can be efficiently sorted. Mergesort, on the other hand, follows a merge-and-sort technique, dividing the input array into smaller segments, sorting them, and merging them back together in sorted order.
The time complexity of quicksort and mergesort is typically O(n log n), where n represents the number of elements in the input array. This logarithmic time complexity signifies that the sorting operation's efficiency scales well even as the dataset size increases. This characteristic makes these algorithms suitable for sorting large datasets, ensuring fast and reliable performance.
Flexibility
The flexibility offered by Java's sorting capabilities is deeply intertwined with "sort in descending order java" because it enables the sorting operation to be applied to various data structures, including arrays and lists.
In the context of real-life applications, this flexibility is crucial because different data structures are suited for different scenarios. For example, arrays provide efficient random access, while linked lists excel in insertion and deletion operations. By supporting sorting across these data structures, Java empowers developers to choose the most appropriate structure for their specific requirements, ensuring optimal performance and code maintainability.
Furthermore, the ability to sort custom objects by implementing the Comparable interface or using lambda expressions with the sort() method enhances the versatility of "sort in descending order java." This feature allows developers to define custom sorting criteria tailored to their specific domain or problem, adding to the overall power and adaptability of the sorting functionality.
Data Analysis
In the realm of data analysis, sorting in descending order plays a vital role in uncovering valuable insights and patterns within datasets. By arranging data in decreasing order, analysts gain a clear perspective of the highest values, extreme values (outliers), and overall trends.
- Maximum Value Identification: Descending order sorting allows for the swift identification of the maximum value within a dataset. This is particularly useful in scenarios where the largest value represents a critical metric, such as the highest sales revenue or the maximum temperature recorded.
- Outlier Detection: Outliers are data points that significantly deviate from the rest of the data. Sorting in descending order helps analysts easily spot these extreme values, which may indicate anomalies, errors, or exceptional cases that require further investigation.
- Trend Analysis: When data is sorted in descending order, analysts can observe the gradual change in values. This facilitates the identification of trends, patterns, and correlations within the data. By examining the sequence of values, analysts can gain insights into how a particular metric has evolved over time or how different variables relate to each other.
The ability to sort data in descending order is thus an essential tool for data analysts, empowering them to uncover meaningful insights, make informed decisions, and gain a deeper understanding of the data at hand.
Optimization
In the realm of computer science, optimization techniques play a pivotal role in enhancing the efficiency and performance of various applications. Sorting in descending order, denoted by "sort in descending order java," serves as a crucial component of optimization, particularly in the context of priority queues.
A priority queue is a specialized data structure that stores and retrieves elements based on their priority, typically implemented using a heap data structure. Sorting in descending order becomes essential in this scenario to ensure that the element with the highest priority is retrieved first, enabling efficient retrieval of the largest elements.
Consider a real-life example of a hospital emergency room where patients are triaged based on the severity of their injuries. By sorting the incoming patients in descending order of their medical urgency, the medical staff can swiftly identify and prioritize the patients who require immediate attention. This optimized approach ensures that critical cases are handled promptly, potentially saving lives.
The practical significance of understanding the connection between "sort in descending order java" and optimization lies in its widespread applicability across diverse domains. From managing customer support queues to processing financial transactions, efficient retrieval of the largest elements is essential for optimizing performance and delivering timely and effective services.
In summary, sorting in descending order plays a vital role in optimization, particularly in the context of priority queues and other applications where efficient retrieval of the largest elements is paramount. This understanding empowers developers to design and implement systems that can handle complex scenarios with improved performance and efficiency.
Real-World Applications
Sorting in descending order plays a crucial role in numerous real-world applications across diverse domains. Its ability to organize data in decreasing order provides valuable insights and optimizes performance in various scenarios.
- Finance:
In the financial sector, descending order sorting is essential for tasks such as identifying the largest shareholders, tracking stock prices over time, and analyzing market trends. By arranging financial data in descending order, analysts can quickly pinpoint key metrics and make informed decisions.
- Inventory Management:
Descending order sorting is vital in inventory management systems. It allows businesses to identify products with the highest demand, track inventory levels, and prioritize restocking. By organizing inventory data in descending order, businesses can optimize their stock levels and minimize the risk of stockouts.
- Scientific Computing:
In scientific computing, descending order sorting is used to analyze large datasets, identify patterns, and perform complex simulations. It helps scientists arrange data in a meaningful order, enabling them to draw valuable conclusions and make informed predictions.
These examples illustrate the practical significance of "sort in descending order java" and its wide-ranging applications. By understanding the connection between sorting in descending order and real-world scenarios, developers can leverage this technique to create efficient and effective solutions.
FAQs about "sort in descending order java"
This section addresses common questions and misconceptions surrounding "sort in descending order java" to provide a comprehensive understanding of the topic.
Question 1: What are the benefits of sorting in descending order in Java?
Answer: Sorting in descending order offers several advantages, including easy identification of maximum values, detection of outliers, efficient retrieval of largest elements, and trend analysis in datasets.
Question 2: How do I sort an array in descending order in Java?
Answer: To sort an array in descending order in Java, you can use the sort() method along with the Collections.reverseOrder() comparator. This method arranges the elements in decreasing order, with the largest element at the beginning of the array.
Question 3: Is sorting in descending order efficient in Java?
Answer: Yes, sorting in descending order in Java is efficient. Java utilizes sorting algorithms like quicksort and mergesort, which have a time complexity of O(n log n), ensuring efficient sorting even for large datasets.
Question 4: Can I sort custom objects in descending order in Java?
Answer: Yes, you can sort custom objects in descending order in Java by implementing the Comparable interface or using lambda expressions with the sort() method. This allows you to define custom sorting criteria based on specific properties of your objects.
Question 5: What are some real-world applications of sorting in descending order?
Answer: Sorting in descending order finds applications in various domains, such as finance (identifying largest shareholders), inventory management (tracking stock levels), and scientific computing (analyzing large datasets).
Question 6: How can I improve the performance of sorting in descending order in Java?
Answer: To enhance the performance of sorting in descending order in Java, consider using efficient data structures like arrays or linked lists, optimizing the sorting algorithm based on the specific dataset, and leveraging multithreading techniques for parallel sorting.
These FAQs provide a comprehensive overview of "sort in descending order java," clarifying common doubts and offering practical guidance for effective implementation.
Transition to the next article section:Moving beyond the basics of sorting in descending order, let's delve into advanced techniques and explore the intricacies of sorting algorithms and optimization strategies.
Conclusion
Sorting in descending order in Java is a fundamental technique with wide-ranging applications. Its simplicity, efficiency, and flexibility make it a valuable tool for organizing and analyzing data. Whether you are working with arrays, lists, or custom objects, Java provides robust methods to sort data in descending order, empowering developers to uncover insights, optimize performance, and solve complex problems.
Beyond the basics covered in this article, there are advanced techniques and optimizations that can further enhance the efficiency and effectiveness of sorting algorithms. As you delve deeper into the world of sorting, you will discover a rich and dynamic field with ongoing research and innovative approaches. Embrace the opportunity to explore these advanced concepts and push the boundaries of your knowledge.
The Ultimate Guide To All Freddy Characters: Unlocking The FNAF Universe
Maximizing Efficiency: Understanding Productive Vs. Allocative Efficiency
Ultimate Guide To Oklahoma Homestead Exemptions: Protect Your Home From Creditors

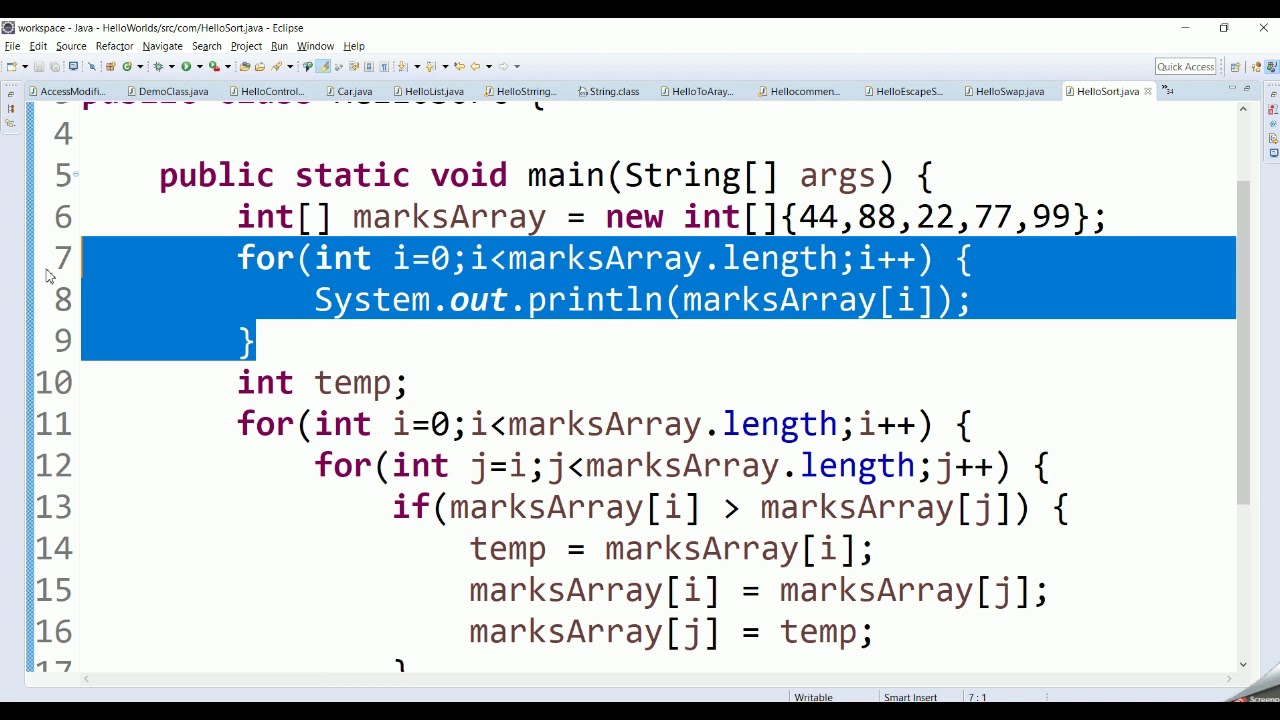
